Running Tasks & Console
It can be common for Rails engineers to fire up the Rails console for some quick debugging or to run code like a Rake task. That said, console'ing into a Lambda function (typically done via SSH) is not possible and requires an event-driven & stateless solution. For this, we have the Lambda Console tool.
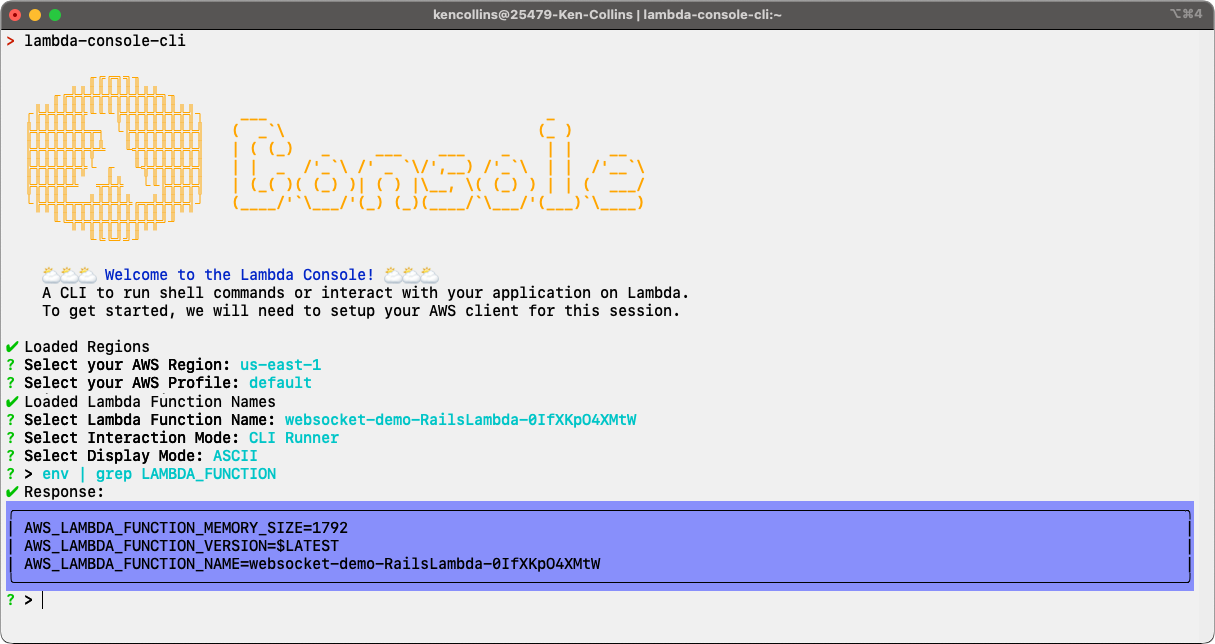
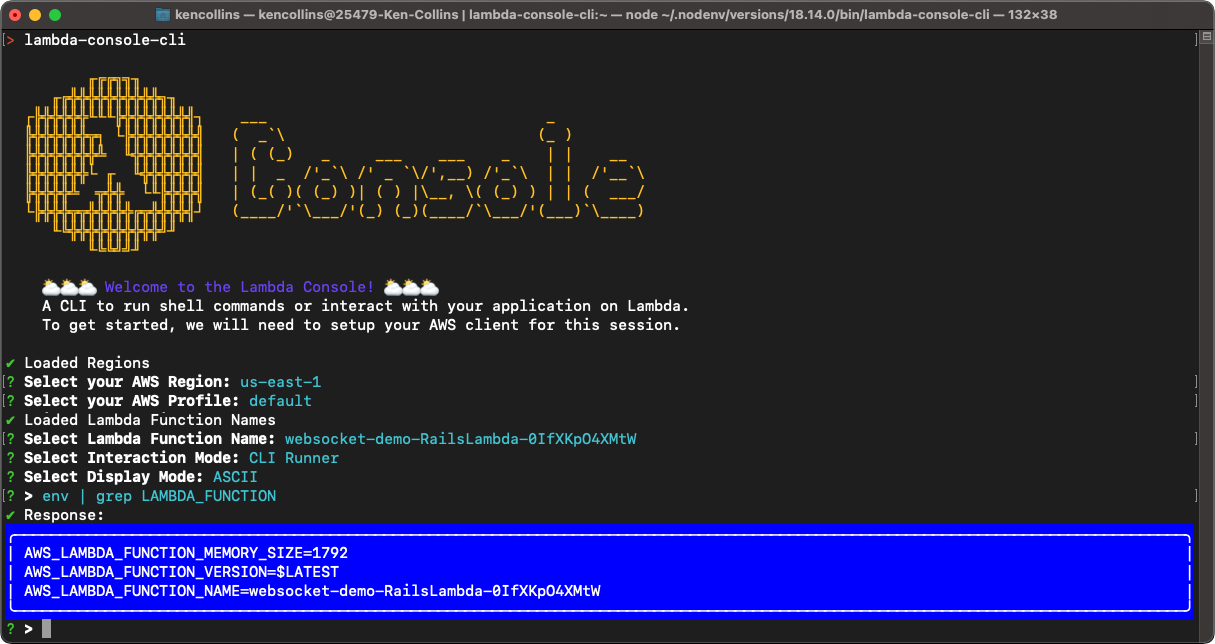
💁♂️ https://github.com/rails-lambda/lambda-console
Lamby leverages the Lambda Console using our Ruby implementation of the spec via the lambda-console-ruby gem. Here is a quick overview on how to use it for common Rails tasks. Please see the Lambda Console project for complete documentation on the CLI installation and usage.
To use the Lambda Console, please make sure you are using Lamby v5.0.0 or higher.
Common Considerations
Here are some common considerations when using the Lambda Console to run tasks or interactive commands.
Function Timeout
Each run
or interact
event sent will need to respond within your function's timeout. Since HTTP interactions via most AWS services are limited to 30s, so too is your function's default timeout set to that. If your task takes longer than this, consider temporarily increasing the value in your Cloud Formation template or duplicating your function (copy paste) to a new Lambda Function resource dedicated for running console tasks. A Lambda function can have a maximum of 15m execution time. Just remember that API Gateway integration will always be limited to 30s under the function's timeout. So these timeouts can operate independently.
IAM Security & Permissions
The Lambda Console leverages AWS SDKs to send invoke events to your function(s). This means you are in full control of the security of your function and whom can invoke it with the following IAM actions for your user or role:
lambda:ListFunctions
lambda:InvokeFunction
Customizing Runner Patterns
By default, Lamby v5 and higher allows any command to be run. If you want to enforce which commands can be run at the application layer, please use the Lamby config in your production.rb
environment file.
config.lamby.runner_patterns.clear
config.lamby.runner_patterns.push %r{\A/bin/foo.*}
Here are are clearning/removing the deafault expression pattern of /.*/
in favor of one that allows any /bin/foo
command to be run.